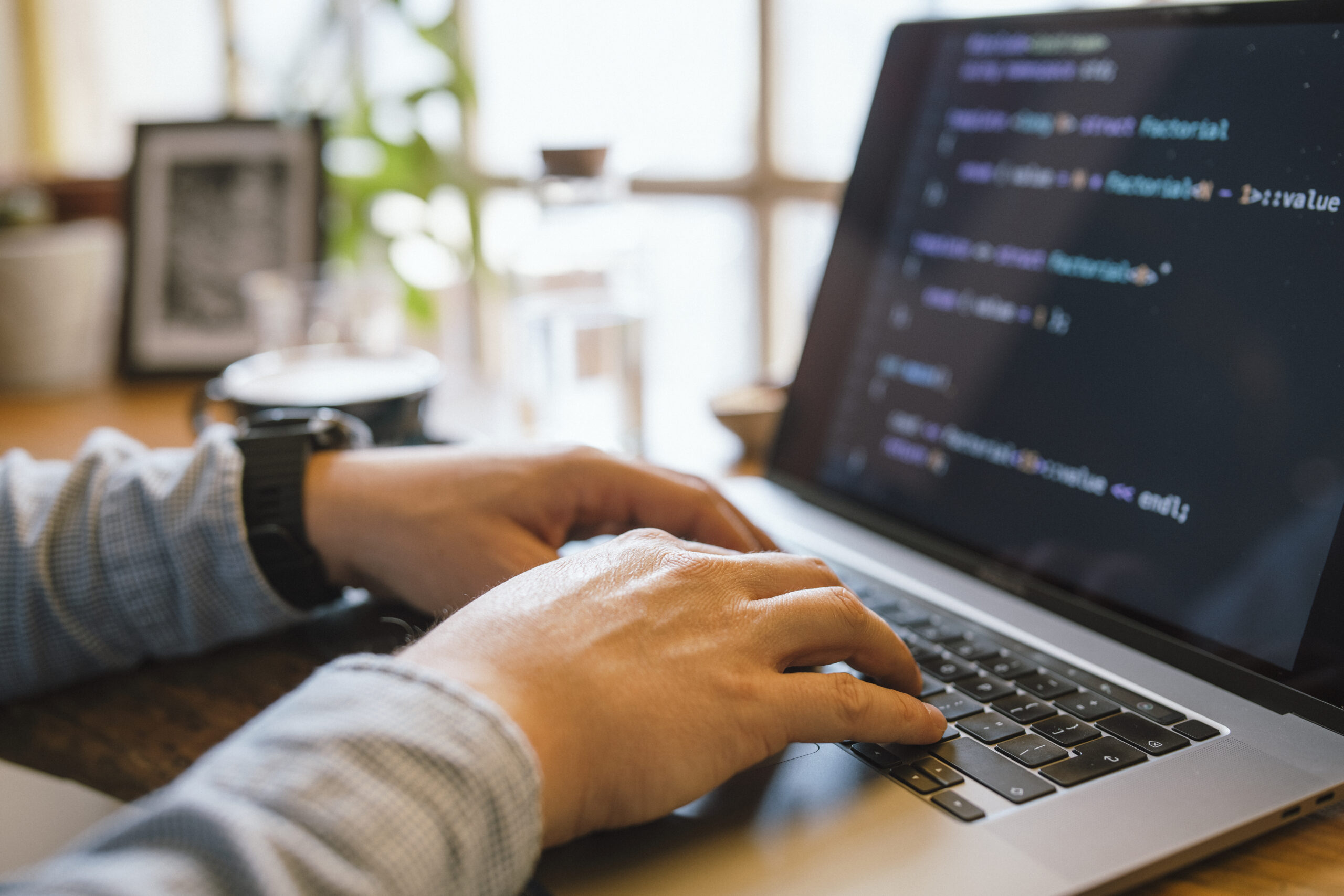
Debugging is Probably the most vital — yet frequently disregarded — capabilities in a very developer’s toolkit. It isn't really just about fixing broken code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies builders can elevate their debugging expertise is by mastering the resources they use every day. While crafting code is one particular A part of development, recognizing tips on how to communicate with it effectively all through execution is Similarly significant. Modern day improvement environments occur Outfitted with powerful debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, as an example, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the worth of variables at runtime, stage via code line by line, and in some cases modify code about the fly. When utilized the right way, they let you observe particularly how your code behaves throughout execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer tools, including Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, check network requests, see true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert frustrating UI challenges into manageable jobs.
For backend or procedure-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Understanding these instruments could have a steeper Discovering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with Variation control methods like Git to grasp code heritage, obtain the precise moment bugs ended up released, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement atmosphere in order that when concerns crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you could invest solving the particular trouble rather than fumbling through the procedure.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the situation. Right before leaping to the code or producing guesses, developers have to have to produce a regular natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a game of likelihood, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering as much context as is possible. Request concerns like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact circumstances underneath which the bug occurs.
As you’ve collected more than enough details, try to recreate the condition in your local ecosystem. This could necessarily mean inputting the identical details, simulating equivalent user interactions, or mimicking technique states. If the issue appears intermittently, take into consideration creating automatic checks that replicate the edge cases or state transitions included. These checks not just enable expose the issue but in addition stop regressions Sooner or later.
Sometimes, the issue could possibly be environment-certain — it would occur only on specified functioning systems, browsers, or below distinct configurations. Applying resources like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to repairing it. Using a reproducible circumstance, You should utilize your debugging instruments additional correctly, exam potential fixes securely, and talk a lot more Obviously along with your group or consumers. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages in many cases are the most useful clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers ought to discover to take care of mistake messages as direct communications in the system. They normally tell you what precisely took place, in which it happened, and sometimes even why it took place — if you understand how to interpret them.
Begin by reading through the message thoroughly and in full. Quite a few developers, specially when underneath time force, glance at the main line and promptly commence making assumptions. But further within the mistake stack or logs may well lie the correct root cause. Don’t just duplicate and paste mistake messages into serps — go through and understand them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a selected file and line amount? What module or functionality induced it? These thoughts can manual your investigation and issue you toward the dependable code.
It’s also helpful to be familiar with the terminology in the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically abide by predictable designs, and Mastering to recognize these can considerably quicken your debugging approach.
Some faults are vague or generic, and in All those cases, it’s critical to examine the context during which the mistake happened. Verify connected log entries, enter values, and up to date changes inside the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and assured developer.
Use Logging Properly
Logging is The most strong instruments in a very developer’s debugging toolkit. When made use of effectively, it offers serious-time insights into how an application behaves, aiding you realize what’s taking place under the hood with no need to pause execution or stage with the code line by line.
A great logging approach begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common occasions (like successful get started-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your system. Deal with essential gatherings, condition changes, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and regularly. Involve context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you monitor how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your applications, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a form of investigation. To efficiently establish and take care of bugs, developers should technique the method similar to a detective resolving a secret. This frame of mind can help stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by collecting evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain as much related info as you are able to without having jumping to conclusions. Use logs, test instances, and user reports to piece together a clear picture of what’s happening.
Future, variety hypotheses. Talk to on your own: What may very well be resulting in this habits? Have any adjustments not long ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The aim would be to slender down possibilities and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a controlled atmosphere. For those who suspect a certain purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Spend shut focus to tiny details. Bugs normally cover within the the very least predicted spots—just like a lacking semicolon, an off-by-one particular error, or a race issue. Be complete and individual, resisting the urge to patch the issue without entirely understanding it. Temporary fixes may cover the actual issue, just for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Just as detectives log their investigations, documenting your debugging course of action can preserve time for future problems and enable Other individuals comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution challenges methodically, and turn into more practical at uncovering hidden difficulties in complex methods.
Compose Assessments
Crafting checks is one of the best solutions to improve your debugging abilities and All round progress efficiency. Tests not just support capture bugs early and also function a safety Internet that provides you assurance when making modifications in your codebase. A properly-examined application is simpler to debug as it means that you can pinpoint precisely exactly where and when a difficulty happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can swiftly expose no matter if a certain bit of logic is Doing work as anticipated. Each time a check fails, you right away know in which to search, substantially decreasing enough time put in debugging. Unit tests are especially helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Subsequent, combine integration tests and close-to-close assessments into your workflow. These aid be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that take place in complicated units with a number of elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline failed and beneath what circumstances.
Crafting checks also forces you to Imagine critically regarding your code. To test a element correctly, you would like to grasp its inputs, expected outputs, and edge situations. This degree of being familiar with By natural means prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug could be a robust first step. After the exam fails constantly, you could center on fixing the bug and look at your exam pass when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, composing assessments turns debugging from the discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—watching your display screen for several hours, seeking solution following Answer. But The most underrated debugging instruments is actually stepping absent. Getting breaks allows you reset your intellect, reduce stress, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could start off overlooking clear problems or misreading code that you just wrote just hours before. During this point out, your Mind will become considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different process for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance prevent burnout, Specifically throughout for a longer period debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do here a thing unrelated to code. It may sense counterintuitive, Particularly less than restricted deadlines, but it really truly causes more quickly and more practical debugging Eventually.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a wise tactic. It gives your brain House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a psychological puzzle, and rest is part of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, every one can teach you some thing worthwhile when you take the time to reflect and analyze what went Improper.
Commence by inquiring by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught previously with superior techniques like device screening, code opinions, or logging? The solutions generally expose blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively keep away from.
In group environments, sharing what you've acquired from the bug with the peers may be especially impressive. No matter if it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, assisting others stay away from the identical issue boosts staff efficiency and cultivates a much better Understanding tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously understand from their mistakes.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques requires time, follow, and tolerance — but the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.